Android and OkHttp (HTTP LIB:Library) อีก Library ตัวหนึ่งในการเขียน Android App ที่น่าสนใจไว้สำหรับการจัดการในการเชื่อมต่อกับ Http คือ OkHttp โดยไลบรารี่ตัวนี้ค่อนข้างจะทำงานได้ดีในระดับหนึ่ง ความสามารถพื้นฐานรองรับการการรับ-ส่งข้อมูลในรูปแบบ Get และ Post สามารถส่งได้ทั้งที่เป็น String , Multipart File , Streaming และอื่น ๆ อีกหลายฟีเจอร์ สั่งความสามารถพิเศษที่น่าจะสนใจ คือจะใช้ Gson ทั้งการ รับและส่ง ได้เช่นเดียวกัน
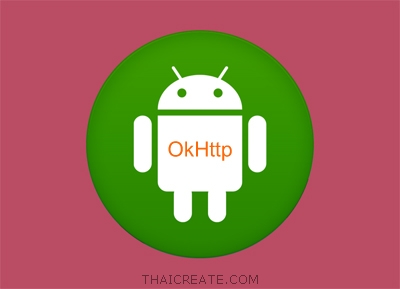
Android and OkHttp
ในการเขียน Android App ช่วงหลัง ๆ ผมจึงแนะนำให้ใช้ Library มาเข้าจัดการกับเรื่องเหล่านี้แทน ซึ่งในปัจจุบันมี Library หลายตัวมาก ที่เข้ามาจัดการกับ Http โดยเฉพาะ รองรับการทำงานเกือบทุกรูปแบบ ไม่ว่าจะเป็น get, post การรับส่ง string , files หรือแม้แต่ json ก็ยังถูกนำมารวมใช้งานกับ library นี้ด้วย โดยที่เราไม่จำเป็นจะต้องใช้การ parser ค่ามันอีก เพราะมันจะแปลงมาเป็น json ให้เลย ทั้ง ไป-กลับ และ function ต่าง ๆ ที่อยู่ใน Library เราจะมั่นใจได้ว่ามันจะไม่ถูก deprecated แน่นอน เพราะถ้ามีการ deprecated เราก็จะสามารถอัพเดดเวอร์ชั่นใหม่ ๆ ได้ตลอด โดยที่ไม่จำเป็นจะต้องมาแก้ไข Code ใหม่
สำหรับตัวอย่างและ Code นี้รองรับการเขียนทั้งบนโปรแกรม Eclipse และ Android Studio
Download OkHttp
การติดตั้งสามารถดาวน์โหลดไฟล์ .JAR แล้วนำไป Import ลงใน Eclipse หรือ Android Studio
ในการใช้งาน OkHttp จะต้องใช้ Library ของ okio ด้วย และจะรองรับ Level 19 หรือ Android 4.4.2 (เวอร์ชั่นต่ำกว่านี้น่าจะได้)
กรณีใช้บน Android Studio เพิ่ม build.gradle
compile ‘com.squareup.okio:okio:1.6.0’
ใน AndroidManifest.xml เพิ่ม Permission สำหรับการเชื่อมต่อกับ Internet ดังนี้
1.
<
uses-permission
android:name
=
"android.permission.INTERNET"
/>
ขั้นตอนการเรียกใช้
Example 1 : การเรียก URL ผ่าน get
Syntax
01.
OkHttpClient client =
new
OkHttpClient();
02.
03.
String run(String url)
throws
IOException {
04.
Request request =
new
Request.Builder()
05.
.url(url)
06.
.build();
07.
08.
Response response = client.newCall(request).execute();
09.
return
response.body().string();
10.
}
getString.php : ตัวอย่างไฟล์ PHP ที่อยู่บน Server (www.thaicreate.com/android/getString.php)
1.
<?php
2.
echo
date
(
"Y-m-d H:i:s"
);
3.
?>
activity_main.xml
01.
<
TableLayout
xmlns:android
=
"http://schemas.android.com/apk/res/android"
02.
xmlns:tools
=
"http://schemas.android.com/tools"
03.
android:layout_width
=
"match_parent"
04.
android:layout_height
=
"match_parent"
>
05.
06.
<
TextView
07.
android:id
=
"@+id/txtResult"
08.
android:layout_width
=
"wrap_content"
09.
android:layout_height
=
"wrap_content"
10.
android:layout_marginTop
=
"50dp"
11.
android:gravity
=
"center"
12.
android:text
=
"Result"
/>
13.
14.
</
TableLayout
>
MainActivity.java
01.
package
com.myapp;
02.
03.
import
android.os.Bundle;
04.
import
android.os.StrictMode;
05.
06.
import
java.io.IOException;
07.
import
com.squareup.okhttp.OkHttpClient;
08.
import
com.squareup.okhttp.Request;
09.
import
com.squareup.okhttp.Response;
10.
11.
import
android.app.Activity;
12.
import
android.widget.TextView;
13.
14.
public
class
MainActivity
extends
Activity {
15.
16.
@Override
17.
public
void
onCreate(Bundle savedInstanceState) {
18.
super
.onCreate(savedInstanceState);
19.
setContentView(R.layout.activity_main);
20.
21.
// Permission StrictMode
22.
if
(android.os.Build.VERSION.SDK_INT >
9
) {
23.
StrictMode.ThreadPolicy policy =
new
StrictMode.ThreadPolicy.Builder().permitAll().build();
24.
StrictMode.setThreadPolicy(policy);
25.
}
26.
27.
final
TextView txtResult = (TextView) findViewById(R.id.txtResult);
28.
29.
getHttp http =
new
getHttp();
30.
String response =
null
;
31.
try
{
32.
response = http.run(
"https://www.thaicreate.com/android/getString.php"
);
33.
}
catch
(IOException e) {
34.
// TODO Auto-generated catch block
35.
e.printStackTrace();
36.
}
37.
txtResult.setText(response);
38.
39.
}
40.
41.
public
class
getHttp {
42.
OkHttpClient client =
new
OkHttpClient();
43.
44.
String run(String url)
throws
IOException {
45.
Request request =
new
Request.Builder()
46.
.url(url)
47.
.build();
48.
Response response = client.newCall(request).execute();
49.
return
response.body().string();
50.
}
51.
}
52.
53.
}
Example 2 : การเรียก URL และส่งค่า Parameters ผ่าน post
Syntax
01.
private
final
OkHttpClient client =
new
OkHttpClient();
02.
03.
public
void
run()
throws
Exception {
04.
RequestBody formBody =
new
FormEncodingBuilder()
05.
.add(
"search"
,
"Jurassic Park"
)
06.
.build();
07.
Request request =
new
Request.Builder()
08.
.url(
"https://en.wikipedia.org/w/index.php"
)
09.
.post(formBody)
10.
.build();
11.
12.
Response response = client.newCall(request).execute();
13.
if
(!response.isSuccessful())
throw
new
IOException(
"Unexpected code "
+ response);
14.
15.
System.out.println(response.body().string());
16.
}
postString.php : ตัวอย่างไฟล์ PHP ที่อยู่บน Server (www.thaicreate.com/android/postString.php)
1.
<?php
2.
echo
"Sawatdee : "
.
$_POST
[
"sName"
].
" "
.
$_POST
[
"sLastName"
];
3.
?>
activity_main.xml
01.
<
RelativeLayout
xmlns:android
=
"http://schemas.android.com/apk/res/android"
02.
xmlns:tools
=
"http://schemas.android.com/tools"
03.
android:layout_width
=
"match_parent"
04.
android:layout_height
=
"match_parent"
>
05.
06.
<
TextView
07.
android:id
=
"@+id/txtResult"
08.
android:layout_width
=
"wrap_content"
09.
android:layout_height
=
"wrap_content"
10.
android:layout_alignParentTop
=
"true"
11.
android:layout_centerHorizontal
=
"true"
12.
android:layout_marginTop
=
"107dp"
13.
android:text
=
"Result"
/>
14.
15.
</
RelativeLayout
>
MainActivity.java
01.
package
com.myapp;
02.
03.
import
android.os.Bundle;
04.
import
android.os.StrictMode;
05.
06.
import
java.io.IOException;
07.
08.
import
com.squareup.okhttp.FormEncodingBuilder;
09.
import
com.squareup.okhttp.OkHttpClient;
10.
import
com.squareup.okhttp.Request;
11.
import
com.squareup.okhttp.RequestBody;
12.
import
com.squareup.okhttp.Response;
13.
14.
import
android.app.Activity;
15.
import
android.widget.TextView;
16.
17.
public
class
MainActivity
extends
Activity {
18.
19.
@Override
20.
public
void
onCreate(Bundle savedInstanceState) {
21.
super
.onCreate(savedInstanceState);
22.
setContentView(R.layout.activity_main);
23.
24.
// Permission StrictMode
25.
if
(android.os.Build.VERSION.SDK_INT >
9
) {
26.
StrictMode.ThreadPolicy policy =
new
StrictMode.ThreadPolicy.Builder().permitAll().build();
27.
StrictMode.setThreadPolicy(policy);
28.
}
29.
30.
final
TextView txtResult = (TextView) findViewById(R.id.txtResult);
31.
32.
postHttp http =
new
postHttp();
33.
34.
RequestBody formBody =
new
FormEncodingBuilder()
35.
.add(
"sName"
,
"Weerachai"
)
36.
.add(
"sLastName"
,
"Nukitram"
)
37.
.build();
38.
39.
String response =
null
;
40.
try
{
41.
response = http.run(
"https://www.thaicreate.com/android/postString.php"
,formBody);
42.
}
catch
(IOException e) {
43.
// TODO Auto-generated catch block
44.
e.printStackTrace();
45.
}
46.
txtResult.setText(response);
47.
48.
}
49.
50.
public
class
postHttp {
51.
OkHttpClient client =
new
OkHttpClient();
52.
53.
String run(String url,RequestBody body)
throws
IOException {
54.
Request request =
new
Request.Builder()
55.
.url(url)
56.
.post(body)
57.
.build();
58.
Response response = client.newCall(request).execute();
59.
return
response.body().string();
60.
}
61.
}
62.
63.
}
Example 3 : การส่งค่า Post ไปยัง Web Server พร้อมกับ multipart/form-data
01.
public
static
final
MediaType MEDIA_TYPE_MARKDOWN = MediaType.parse(
"text/x-markdown; charset=utf-8"
);
02.
03.
private
final
OkHttpClient client =
new
OkHttpClient();
04.
05.
public
void
run()
throws
Exception {
06.
File file =
new
File(
"/mnt/README.md"
);
07.
08.
Request request =
new
Request.Builder()
09.
.url(
"https://api.github.com/markdown/raw"
)
10.
.post(RequestBody.create(MEDIA_TYPE_MARKDOWN, file))
11.
.build();
12.
13.
Response response = client.newCall(request).execute();
14.
if
(!response.isSuccessful())
throw
new
IOException(
"Unexpected code "
+ response);
15.
16.
System.out.println(response.body().string());
17.
}
Example 4 : การส่งค่าไปกับ Header
01.
private
final
OkHttpClient client =
new
OkHttpClient();
02.
03.
public
void
run()
throws
Exception {
04.
Request request =
new
Request.Builder()
06.
.header(
"User-Agent"
,
"OkHttp Headers.java"
)
07.
.addHeader(
"Accept"
,
"application/json; q=0.5"
)
08.
.addHeader(
"Accept"
,
"application/vnd.github.v3+json"
)
09.
.build();
10.
11.
Response response = client.newCall(request).execute();
12.
if
(!response.isSuccessful())
throw
new
IOException(
"Unexpected code "
+ response);
13.
14.
System.out.println(
"Server: "
+ response.header(
"Server"
));
15.
System.out.println(
"Date: "
+ response.header(
"Date"
));
16.
System.out.println(
"Vary: "
+ response.headers(
"Vary"
));
17.
}
Example 5 : การส่งค่า Post แบบ JSON (Gson)
01.
private
final
OkHttpClient client =
new
OkHttpClient();
02.
private
final
Gson gson =
new
Gson();
03.
04.
public
void
run()
throws
Exception {
05.
Request request =
new
Request.Builder()
07.
.build();
08.
Response response = client.newCall(request).execute();
09.
if
(!response.isSuccessful())
throw
new
IOException(
"Unexpected code "
+ response);
10.
11.
Gist gist = gson.fromJson(response.body().charStream(), Gist.
class
);
12.
for
(Map.Entry<String, GistFile> entry : gist.files.entrySet()) {
13.
System.out.println(entry.getKey());
14.
System.out.println(entry.getValue().content);
15.
}
16.
}
17.
18.
static
class
Gist {
19.
Map<String, GistFile> files;
20.
}
21.
22.
static
class
GistFile {
23.
String content;
24.
}
ตัวอย่างการใช้งานต่าง ๆ
https://www.thaicreate.com/mobile/android-okhttp.html